Connecting Github Actions across Repositories
I had a request that an iOS swift run
command gets triggered every time a deploy was made to a staging server. This looked like a perfect application for Github Actions. This strategy can be adapted to any CI system.
So here's how I did it.
The iOS Project
I needed to run the command swift run
in a specific directory. Github Actions uses triggers. I decided to use a manual trigger called workflow_dispatch
. Later this will let me trigger it using the API. It also lets me trigger it in the Github UI.
Make a file called .github/workflows/update.yml
(the filename can be whatever you like):
name: Update Graphql
on:
workflow_dispatch
jobs:
build:
name: 'Update Graphql Bits'
runs-on: macOS-latest
steps:
- name: Checkout
users: actions/checkout@master
- name: 'Swift Run'
run: |
cd Codegen
swift run
Some notes:
- The name on the first line matters.
- The tabs/spaces indentation is finicky. Editing this on Github directly is not the worst idea in the world. Their editor gives you feedback. Feedback gives you wings.
We also need to commit if there are any changed files. I used the following:
- name: Add & Commit
uses: EndBug/add-and-commit@v7.0.0
with:
add: 'External/GraphQL'
message: '[auto] Updated GraphQL'
There's a caveat about testing workflow_dispatch
. It only works if it's in your main
branch. So I copied our iOS
project to a fake one, so I could merge to main
and experiment without some git
historian coming in one day and wondering why I the least iOS
capable engineer has made the most commits to the iOS
project.
I ran this a few times in my fake repository to make sure it didn't just keep committing stuff and it worked. Unfortunately, it probably will commit as me until I create my robot account.
Since we proved the concept in our fake repository, we can now move it to the real repository and commit it to the main
branch.
Trigger
The ideal workflow is triggering this command every time we deploy to our staging environment.
name: Staging Deploy
on: deployment_status
jobs:
trigger:
name: Trigger
if: ${{ github.event.deployment.environment == 'staging' && github.event.deployment_status.state == 'success' }}
steps:
- name: Invoke workflow in another repo with inputs
uses: benc-uk/workflow-dispatch@v1
with:
workflow: Update Graphql
repo: alternate/repo
token: ${{ secrets.PERSONAL_TOKEN }}
This action is pretty slick. It takes your token (or your robot's token) and triggers the workflow_dispatch
as an API call.
To test I added this to another fake project. This is my role in life, I create fake projects, fake users, etc, etc. I then used Postman to generate deployments and update the deployment status with the Github API (and using the same PERSONAL_TOKEN
that I use in my action.
This totally worked and was easy. I did run into some debugging issues.
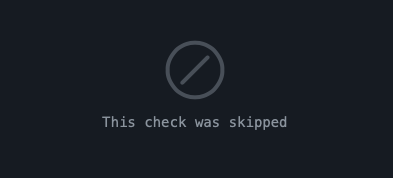
My task kept getting skipped.
I found the Dump Context Action was super valuable for figuring out details of contexts. My issue (which I fixed in the code above) was an error in the if
statement. A million edits later and it finally worked.
In an ideal world I would check 2 extra things:
- That the
sha
that was deployed matched up with the head ofmain
. - That the files affected were related to GraphQL.
But I'm okay with this compromise. My experience is with Jenkins for this type of workload management. I've used Circle CI for smaller operations, but Github Actions was very nice. The marketplace of actions and having this part of Github made this a breeze. Github is already the nexus for so much of our software life-cycle.
Onto the next challenge.
References
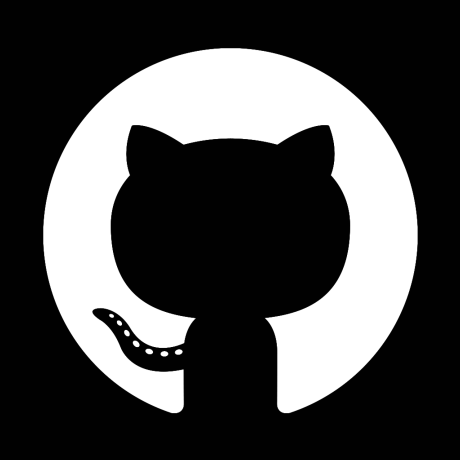
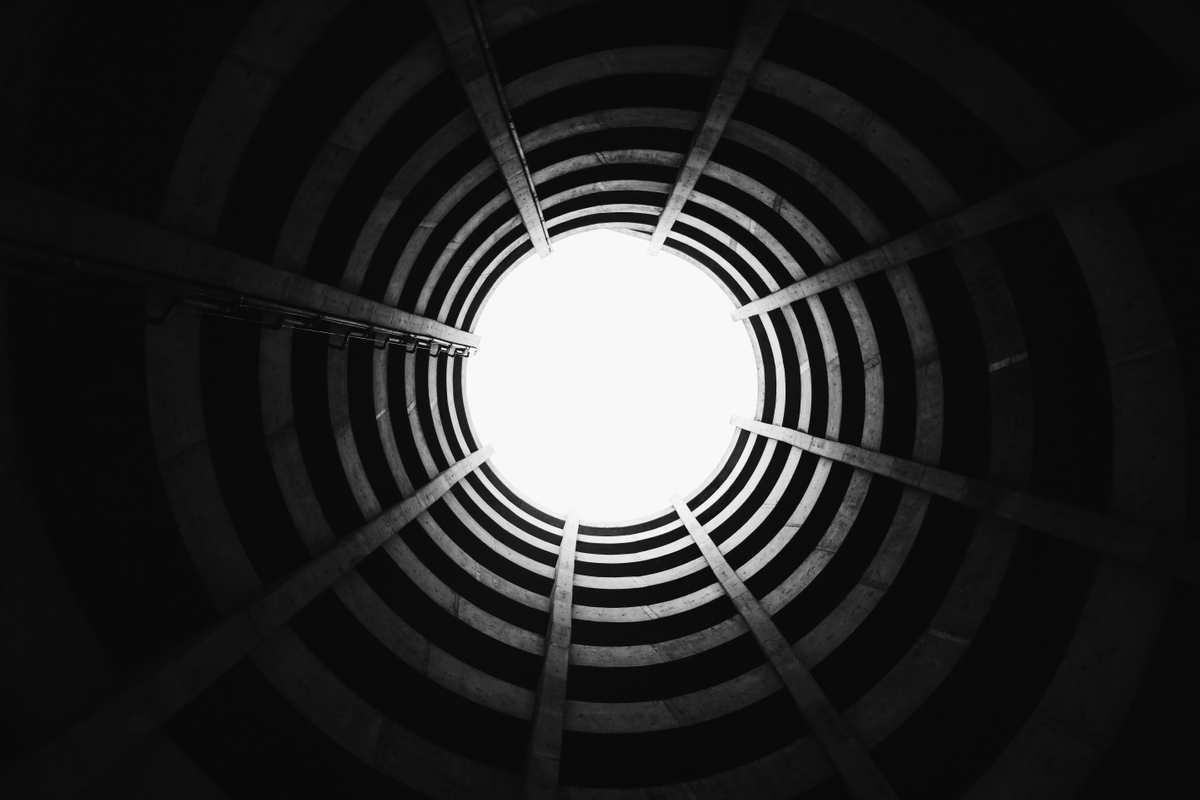
Need help? Ask - I know I left out details, I write this in my spare time and honestly I'm super tired: githubactions@davedash.33mail.com
Want to build Rube-Goldberg machines with me, or make slow things fast, work with me: workwithme@davedash.33mail.com I work at small startup of empathetic engineers and designers.